In the rapidly evolving world of technology, programming has become an essential skill. Whether you’re a beginner or a seasoned coder, there’s always room for improvement. In this article, we’ll explore various strategies to help you improve your programming skills and stay ahead of the curve. This guide is packed with actionable tips, expert advice, and practical exercises designed to take your coding abilities to the next level.
Why Improving Your Programming Skills is Crucial
Table of Contents
Before diving into the strategies, let’s discuss why it’s important to continually improve your programming skills. In the tech industry, change is constant. New programming languages, frameworks, and tools are regularly introduced, making it essential to stay updated. Improving your skills can lead to better job opportunities, higher salaries, and the ability to contribute more effectively to projects.
“Programming isn’t about what you know; it’s about what you can figure out.” — Chris Pine
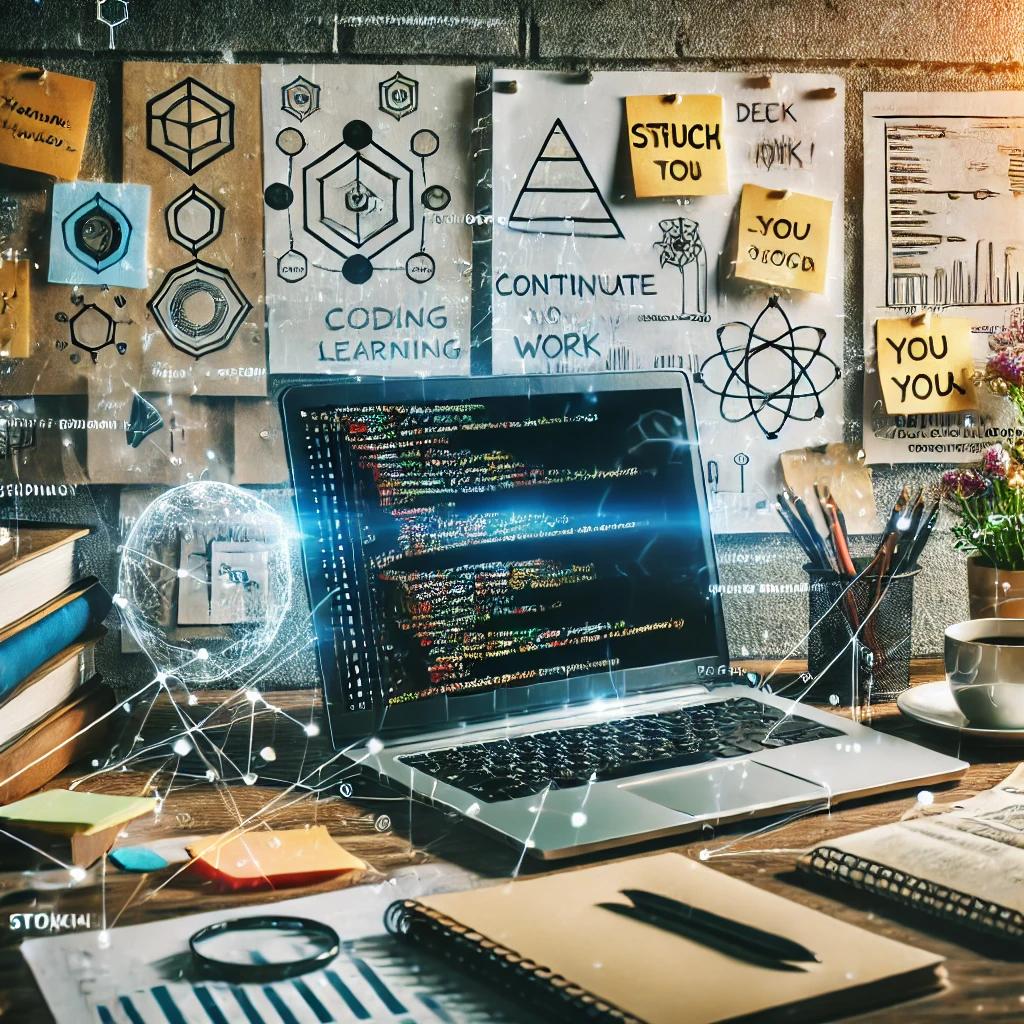
Understanding the Basics: A Strong Foundation is Key
To improve your programming skills, it’s essential to have a solid understanding of the basics. Here’s how you can reinforce your foundational knowledge:
1. Master the Core Concepts
Every programming language has core concepts that are crucial for building complex applications. Focus on mastering:
- Data Structures: Arrays, linked lists, stacks, queues, and trees.
- Algorithms: Sorting, searching, and optimization techniques.
- Object-Oriented Programming (OOP): Classes, objects, inheritance, and polymorphism.
- Control Structures: Loops, conditionals, and switch statements.
2. Practice Problem-Solving
Programming is as much about problem-solving as it is about writing code. Enhance your logical thinking by practicing:
- Coding Challenges: Platforms like LeetCode, HackerRank, and Codewars offer a wide range of problems.
- Math and Logic Puzzles: Engage in puzzles that require analytical thinking.
3. Learn Multiple Programming Languages
While specializing in one language is important, learning multiple languages can broaden your understanding and expose you to different paradigms. For instance:
- Python: Great for beginners and widely used in data science and AI.
- JavaScript: Essential for web development.
- C++: Known for performance and used in system programming.
- Java: Popular for enterprise-level applications.
Developing Advanced Programming Skills
Once you’ve mastered the basics, it’s time to delve into more advanced topics that can significantly improve your programming skills.
4. Explore Data Structures and Algorithms
Advanced data structures and algorithms are critical for efficient coding. Study and implement:
- Graph Algorithms: Depth-first search (DFS), breadth-first search (BFS), Dijkstra’s algorithm.
- Dynamic Programming: Techniques like memoization and tabulation.
- Advanced Data Structures: Heaps, hash tables, and balanced trees.
5. Work on Real-World Projects
Applying your knowledge to real-world projects is one of the most effective ways to learn. Consider:
- Open Source Contributions: Join open-source projects on GitHub to gain practical experience and collaborate with other developers.
- Personal Projects: Build your applications, websites, or tools. This could be anything from a personal blog to a complex data analysis tool.
- Freelancing: Take on freelance projects to challenge yourself with real client requirements.
“The only way to learn a new programming language is by writing programs in it.” — Dennis Ritchie
6. Understand Design Patterns
Design patterns are standard solutions to common problems in software design. Familiarize yourself with:
- Creational Patterns: Singleton, factory, builder.
- Structural Patterns: Adapter, composite, proxy.
- Behavioral Patterns: Observer, strategy, command.
7. Learn About Software Architecture
As you advance in your programming journey, understanding software architecture becomes crucial. Study concepts like:
- MVC (Model-View-Controller): A pattern used in web development.
- Microservices: An architectural style that structures an application as a collection of loosely coupled services.
- Event-Driven Architecture: Focused on events and their handlers to build highly scalable systems.
Enhancing Your Coding Efficiency
Efficiency is key in programming. The faster and more accurately you can write code, the more productive you’ll be. Here are some ways to enhance your coding efficiency:
8. Use Version Control Systems
Version control systems like Git are essential for managing code changes and collaborating with other developers. Learn how to:
- Commit Changes: Regularly commit your work to keep track of your progress.
- Branching and Merging: Work on different features simultaneously and merge them when they’re ready.
- Revert Changes: Roll back to previous versions if something goes wrong.
9. Learn to Use IDEs and Text Editors Effectively
Integrated Development Environments (IDEs) and text editors can significantly boost your productivity. Popular options include:
- Visual Studio Code: A versatile and widely used code editor.
- PyCharm: A powerful IDE for Python development.
- Eclipse: A robust IDE for Java and C++.
10. Automate Repetitive Tasks
Automation can save time and reduce errors. Use scripts to automate tasks like:
- Build Processes: Use tools like Jenkins or Travis CI to automate build and deployment.
- Testing: Automate unit tests with frameworks like JUnit, PyTest, or Jasmine.
- Code Formatting: Tools like Prettier and ESLint can automatically format your code.
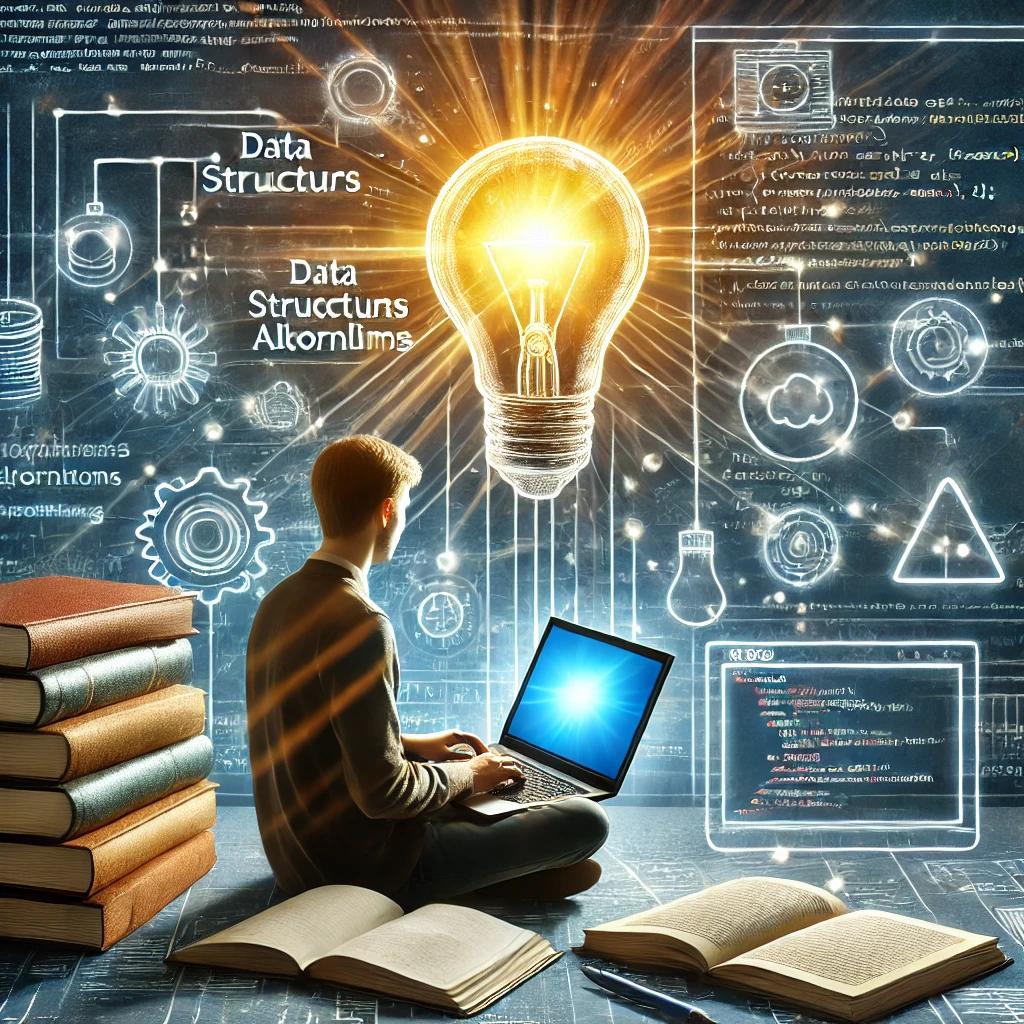
11. Master Debugging Techniques
- Use Debuggers: Learn how to use built-in debuggers in your IDE to step through your code and identify issues.
- Log Outputs: Implement logging to track the flow of your application and catch errors.
- Divide and Conquer: Isolate parts of your code to identify where the problem lies.
“Programs must be written for people to read, and only incidentally for machines to execute.” — Harold Abelson
Debugging is a critical skill for any programmer. To improve your debugging abilities:
Continuous Learning: Staying Updated in the Tech Industry
The tech industry is constantly evolving, and staying updated is key to maintaining and improving your programming skills.
12. Follow Industry Trends
Stay informed about the latest trends in programming by:
- Reading Blogs: Follow popular tech blogs like TechCrunch, Medium, and Stack Overflow.
- Listening to Podcasts: Tune into podcasts like “The Changelog” and “Syntax.”
- Attending Conferences: Participate in industry conferences and webinars to learn from experts.
13. Take Online Courses
Online courses are a great way to learn new programming languages or frameworks. Platforms like:
- Coursera: Offers courses from top universities.
- Udemy: A wide range of courses for all skill levels.
- edX: University-level courses from institutions like MIT and Harvard.
14. Join Coding Communities
Engaging with other programmers can provide valuable insights and support. Join communities like:
- GitHub: Collaborate on projects and share your work.
- Reddit: Participate in programming-related subreddits.
- Stack Overflow: Ask questions and help others in the community.
15. Read Books
Books offer in-depth knowledge and can help you understand complex topics. Some recommended reads include:
- “Clean Code” by Robert C. Martin: A guide to writing clean and maintainable code.
- “The Pragmatic Programmer” by Andrew Hunt and David Thomas: Offers practical advice on becoming a better programmer.
- “Introduction to Algorithms” by Thomas H. Cormen: A comprehensive guide to algorithms.
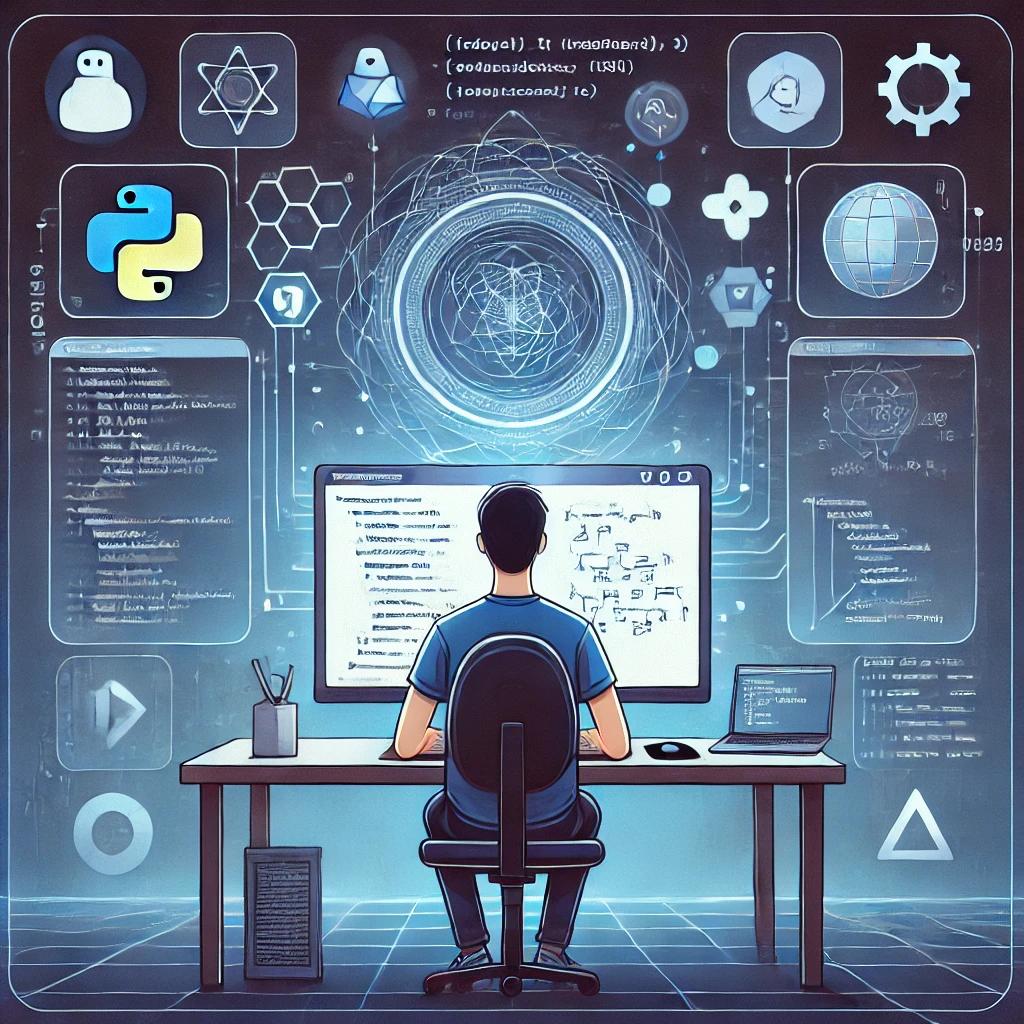
Practical Exercises to Improve Your Programming Skills
Hands-on practice is crucial for improvement. Here are some exercises to help you sharpen your skills:
16. Build a Web Application
Building a web application is a great way to apply your knowledge. Focus on:
- Front-End Development: Learn HTML, CSS, and JavaScript to create interactive user interfaces.
- Back-End Development: Use languages like Python, Ruby, or Node.js to handle server-side logic.
- Databases: Work with SQL or NoSQL databases to store and retrieve data.
17. Contribute to Open Source Projects
Contributing to open-source projects allows you to work on real-world problems and collaborate with other developers. To get started:
- Find a Project: Search for projects on GitHub that match your interests.
- Start Small: Begin with small issues or documentation improvements.
- Engage with the Community: Join discussions and contribute to project planning.
18. Solve Algorithmic Challenges
Algorithmic challenges can improve your problem-solving skills. Platforms like:
- LeetCode: Offers a wide range of coding challenges categorized by difficulty.
- HackerRank: Focuses on algorithmic challenges and competitive programming.
- Project Euler: Provides mathematical problems that require programming solutions.
19. Create a Personal Blog
Sharing your knowledge through a personal blog can reinforce your understanding and establish you as an expert in the field. Topics could include:
- Tutorials: Write about how to solve specific programming problems.
- Case Studies: Share your experiences working on projects.
- Industry Insights: Discuss the latest trends and technologies.
“The best way to learn is by doing. The only way to build a strong work ethic is getting your hands dirty.” — Alex Spanos
20. Practice Code Reviews
Code reviews are essential for learning best practices and improving code quality. You can:
- Review Others’ Code: Participate in code reviews on GitHub or within your team.
- Seek Feedback: Ask for reviews of your code to identify areas for improvement.
- Learn Best Practices: Understand common pitfalls and how to avoid them.
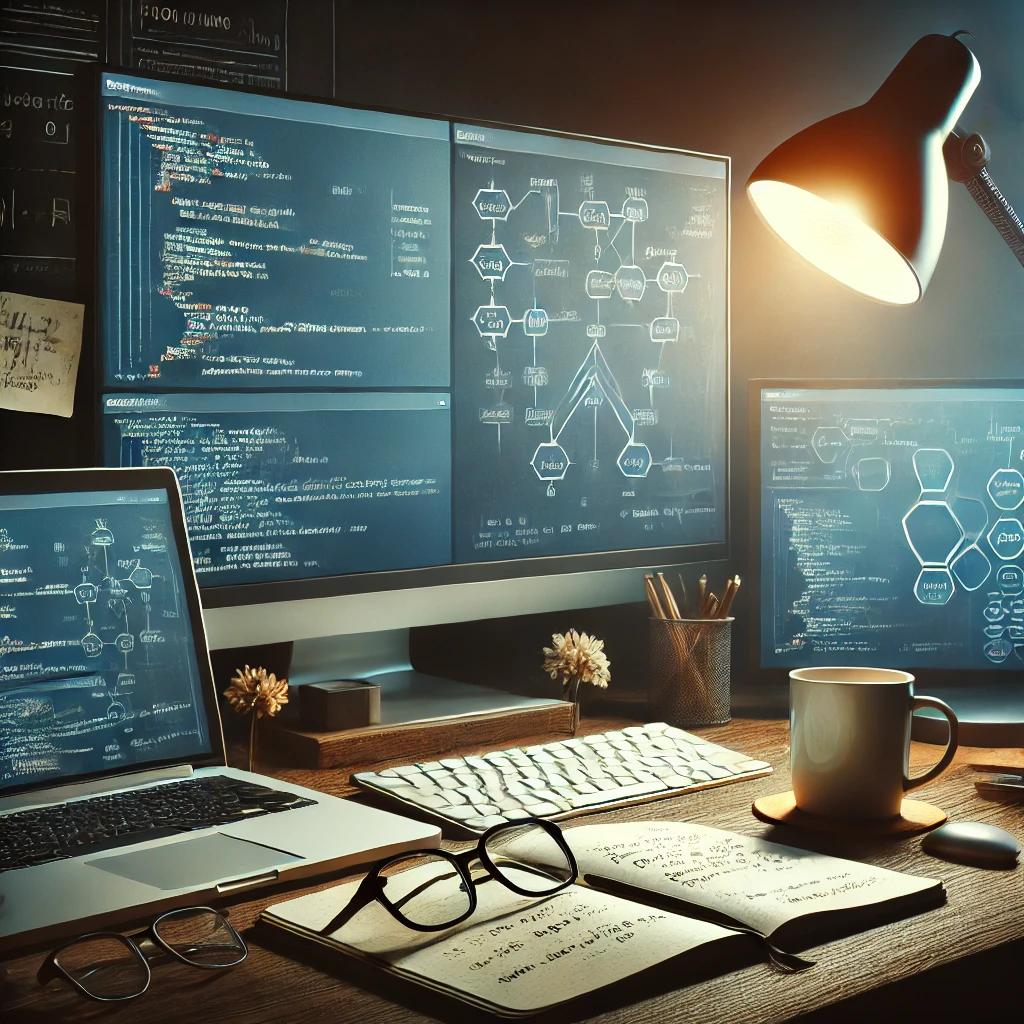
Conclusion: The Path to Mastery in programming skills
Improving your programming skills is a continuous journey that requires dedication, practice, and a willingness to learn. By following the strategies outlined in this article, you can enhance your abilities, stay current with industry trends, and become a more effective and efficient programmer. Remember, the key to success in programming lies in your passion for learning and your persistence in overcoming challenges.
Whether you’re aiming to excel in your current role, transition to a new career, or simply become a better coder, the tips provided here will help you on your journey to mastering the art of programming.
“The more you know, the more you realize you don’t know. The only way to improve is to keep learning.” — Anonymous
Stay curious, keep coding, and never stop improving. Your future self will thank you.
For More articles check the naveedchughtai website.